2.1 入门指南
📝 模块更新日志
-
新特性
-
Serve.IdleHost
支持返回http
和https
协议Web
地址(端口) 4.8.8 ⏱️2023.04.13 fdf7885 -
Serve.GetIdleHost([host])
静态方法,可获取一个指定主机的Web
地址(端口) 4.8.7.43 ⏱️2023.04.12 fdf788 -
Serve.IdleHost
静态属性,可获取一个随机空闲Web
主机地址(端口) 4.8.7.29 ⏱️2023.03.30 e425063 -
WinForm/WPF
静态方法Serve.RunNative()
可配置是否启用Web
主机功能 4.8.7.26 ⏱️2023.03.29 #I6R97L -
WinForm/WPF
快速注册静态方法:Serve.RunNative()
4.8.7.23 ⏱️2023.03.27 53d51c3
-
-
其他更改
-
Serve.Run()
迷你主机默认添加JSON
中文乱码处理 4.8.6.3 ⏱️2023.02.15 86b5f9f
-
-
问题修复
以下内容仅限 Furion 3.6.3 +
版本使用。
Furion
官方提供了非常灵活方便的脚手架,可以快速的创建多层架构项目。
推荐使用 《2.6 官方脚手架》代替本章节功能。
2.1.1 历史背景
相信从 ASP.NET 5
升级至 ASP.NET 6
的开发者都经历过这样变更:
- 在
ASP.NET 5
中,我们这样创建Web 主机
:
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
- 在
ASP.NET 6
中, 我们这样创建Web 主机
:
var builder = WebApplication.CreateBuilder(args);
var app = builder.Build();
试问,ASP.NET 7
,ASP.NET 8
... ASP.NET N
呢?会不会每一个版本都有不同的创建方式,那后续项目如何无缝升级?
所以,为了保证一致的代码体验和后续无缝升级,推出了 Serve.Run()
,即使未来创建方式变了,也不用担心,交给框架即可。
2.1.2 创建 控制台
项目
- 打开
Visual Studio 2022
并创建控 制台
项目
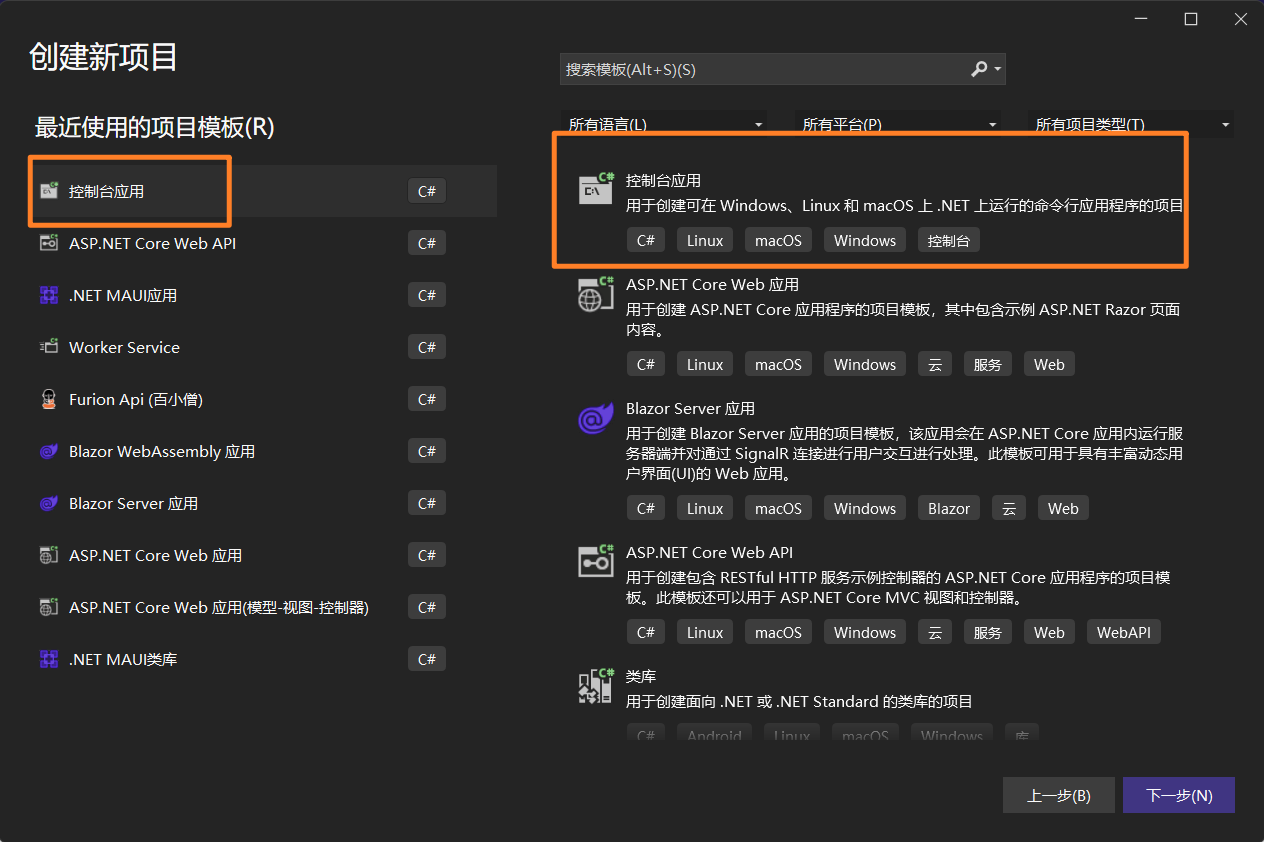
- 配置项目名称
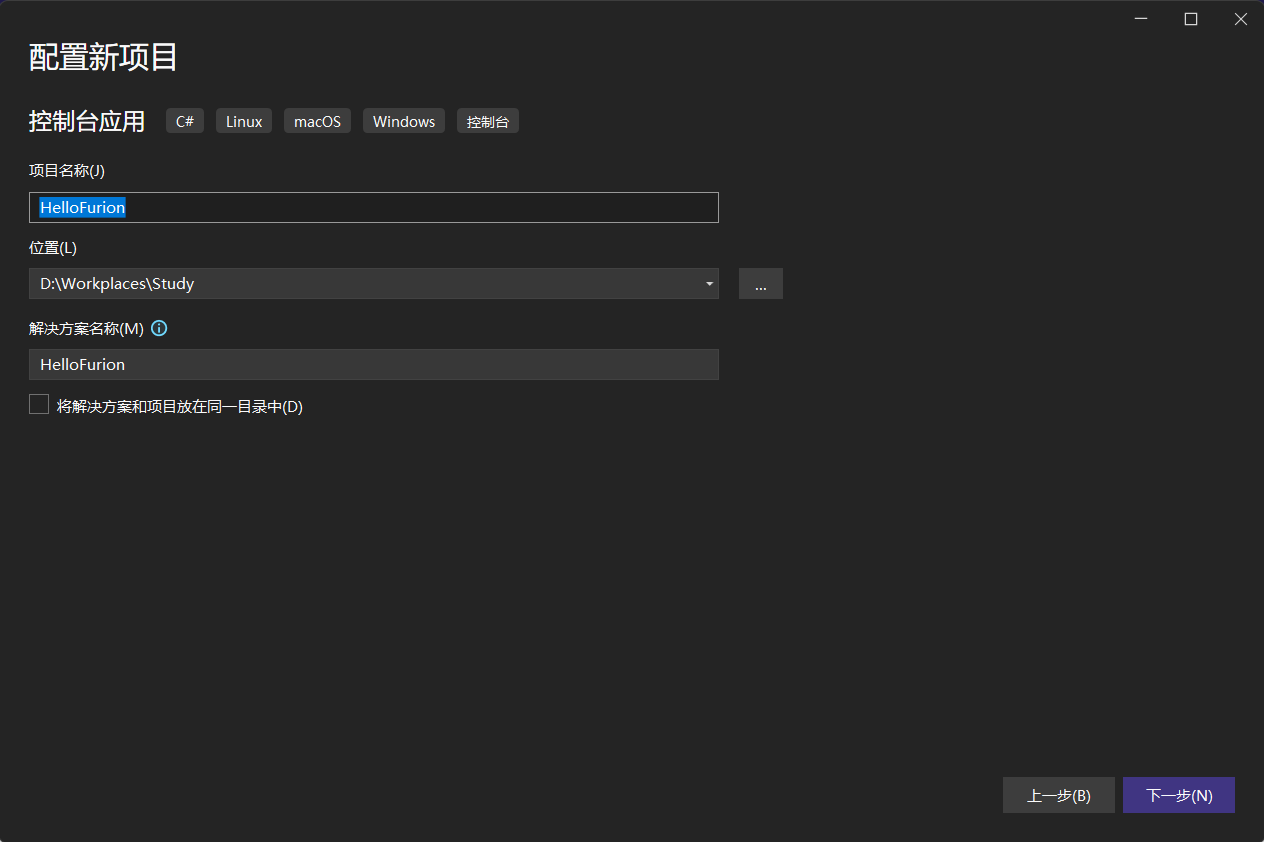
- 选择
.NET6
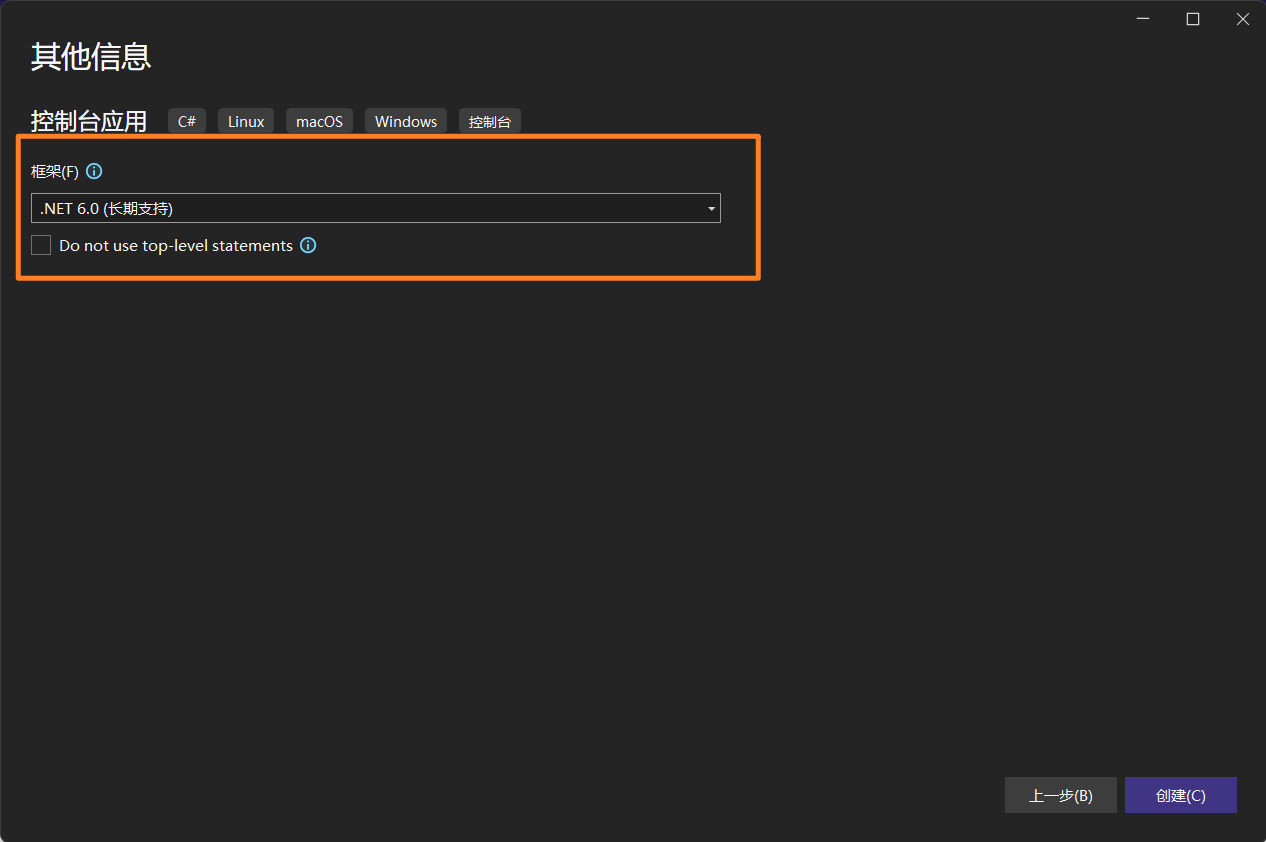
// 创建控制台项目
dotnet new console -n HelloFurion
2.1.3 添加 Furion
依赖包
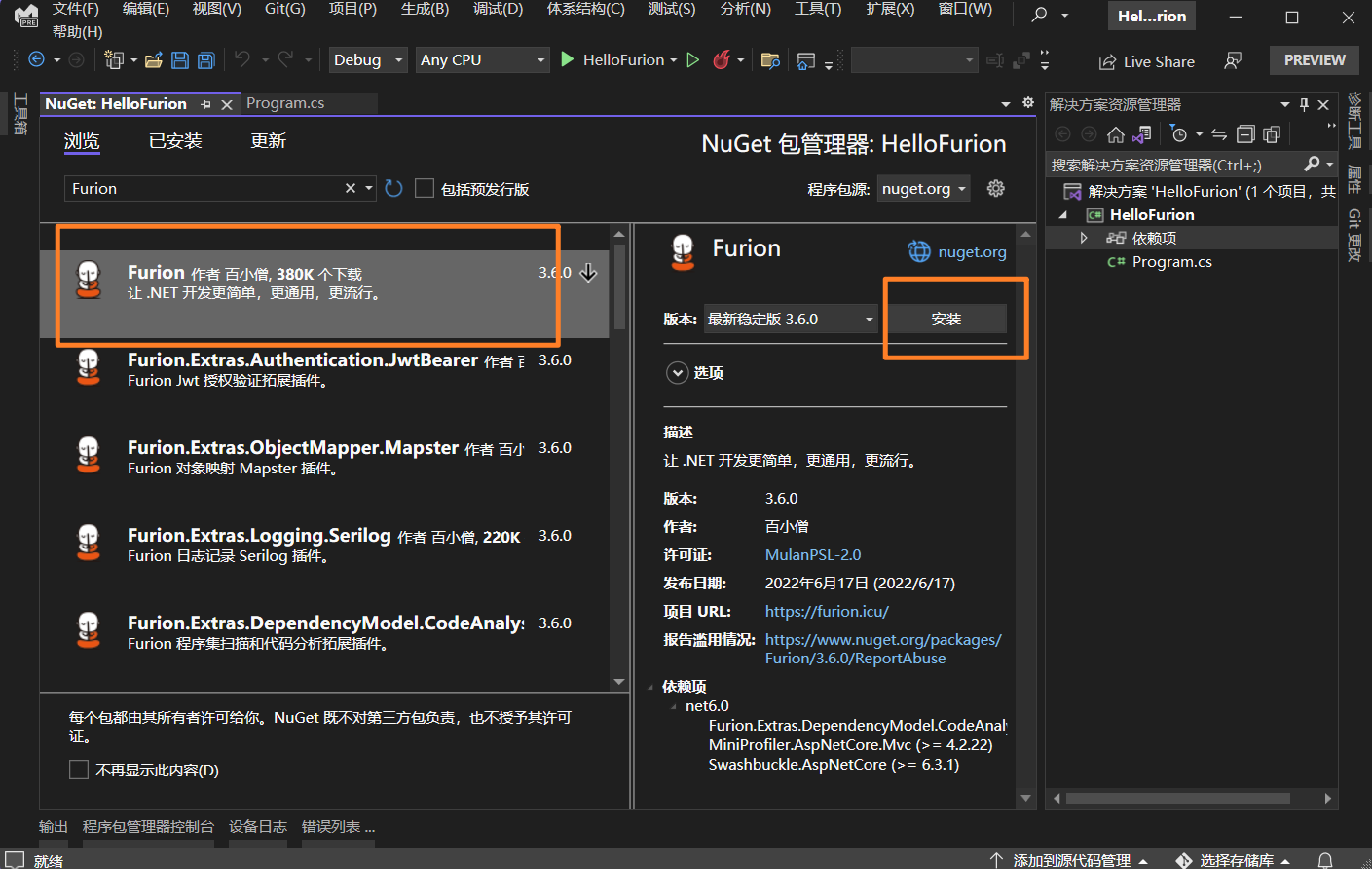
// 进入创建的目录
cd HelloFurion
// 添加包
dotnet add package Furion
2.1.4 一句话搞定
修改 Program.cs
代码为:
Serve.Run();
对,你没看错,Furion
已经配置好了!
Serve.Run()
已经包含了基本的 WebAPI
功能,包含动态 WebAPI
,跨域
等等,如需完全自定义配置可使用 Serve.Run(RunOptions.Default)
,之后 AppStartup
派生类自行配置。
2.1.5 启动浏览器
启动浏览器查看效果
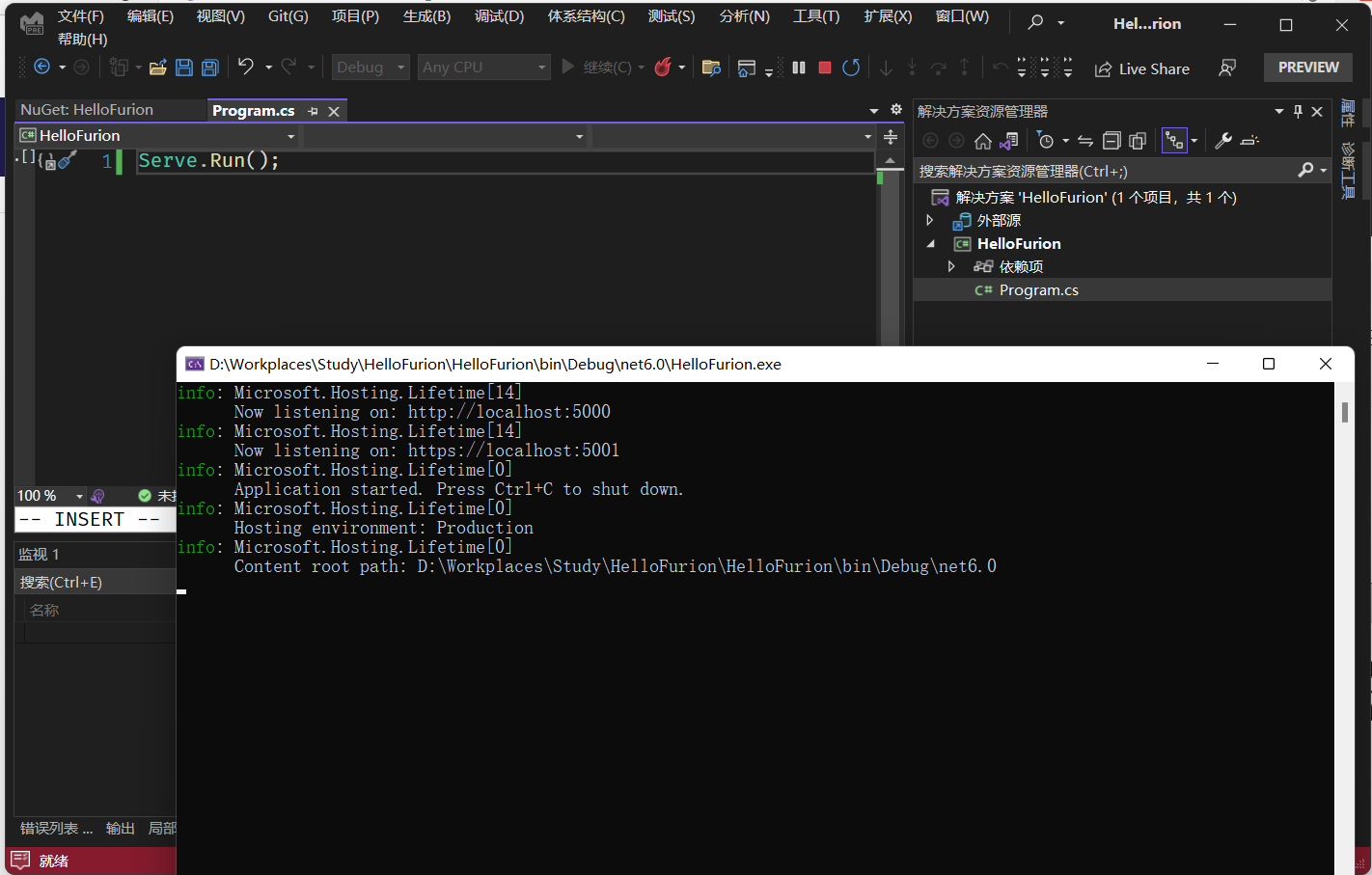
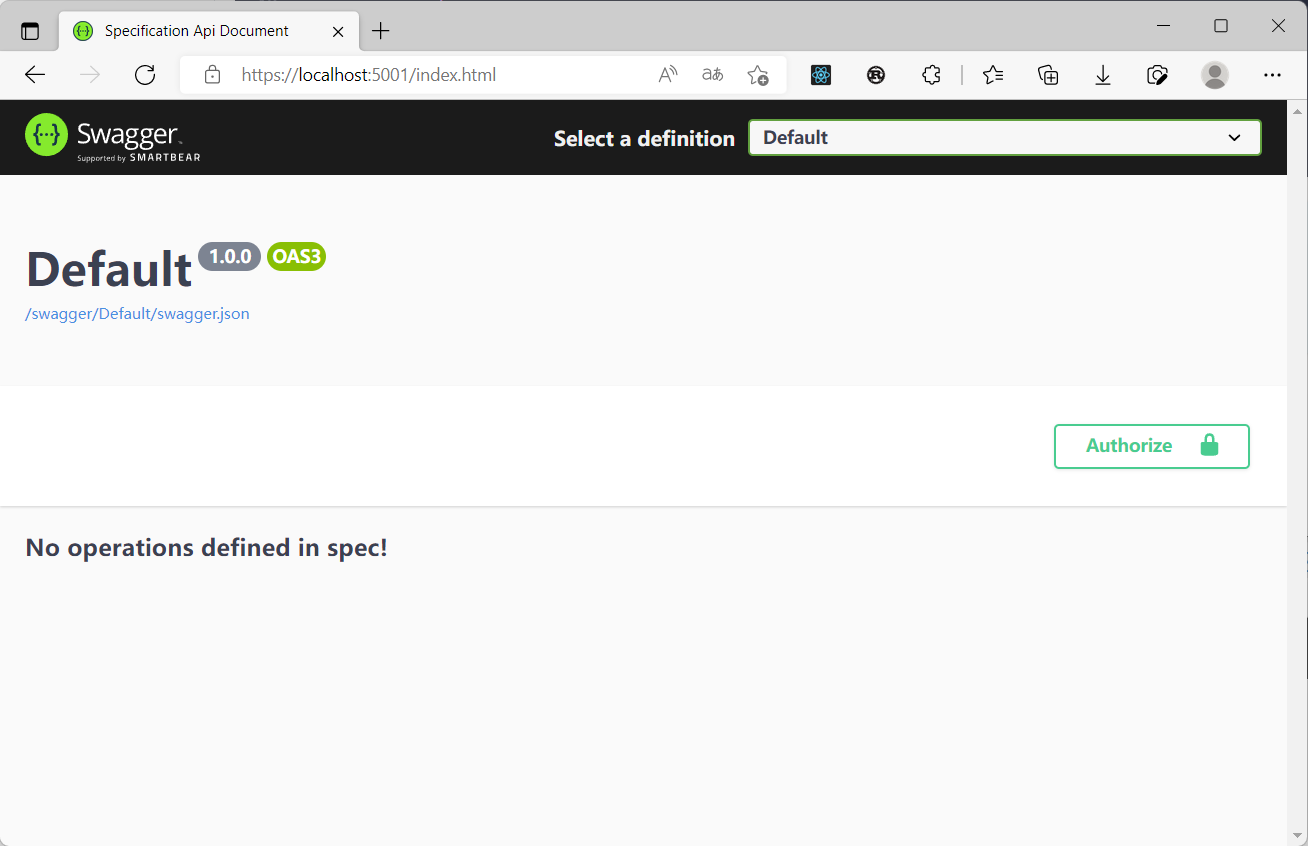
是不是超级超级简单!!!
2.1.6 编写第一个 API
Serve.Run();
[DynamicApiController]
public class HelloService
{
public string Say()
{
return "Hello, Furion";
}
}
启动浏览器查看效果
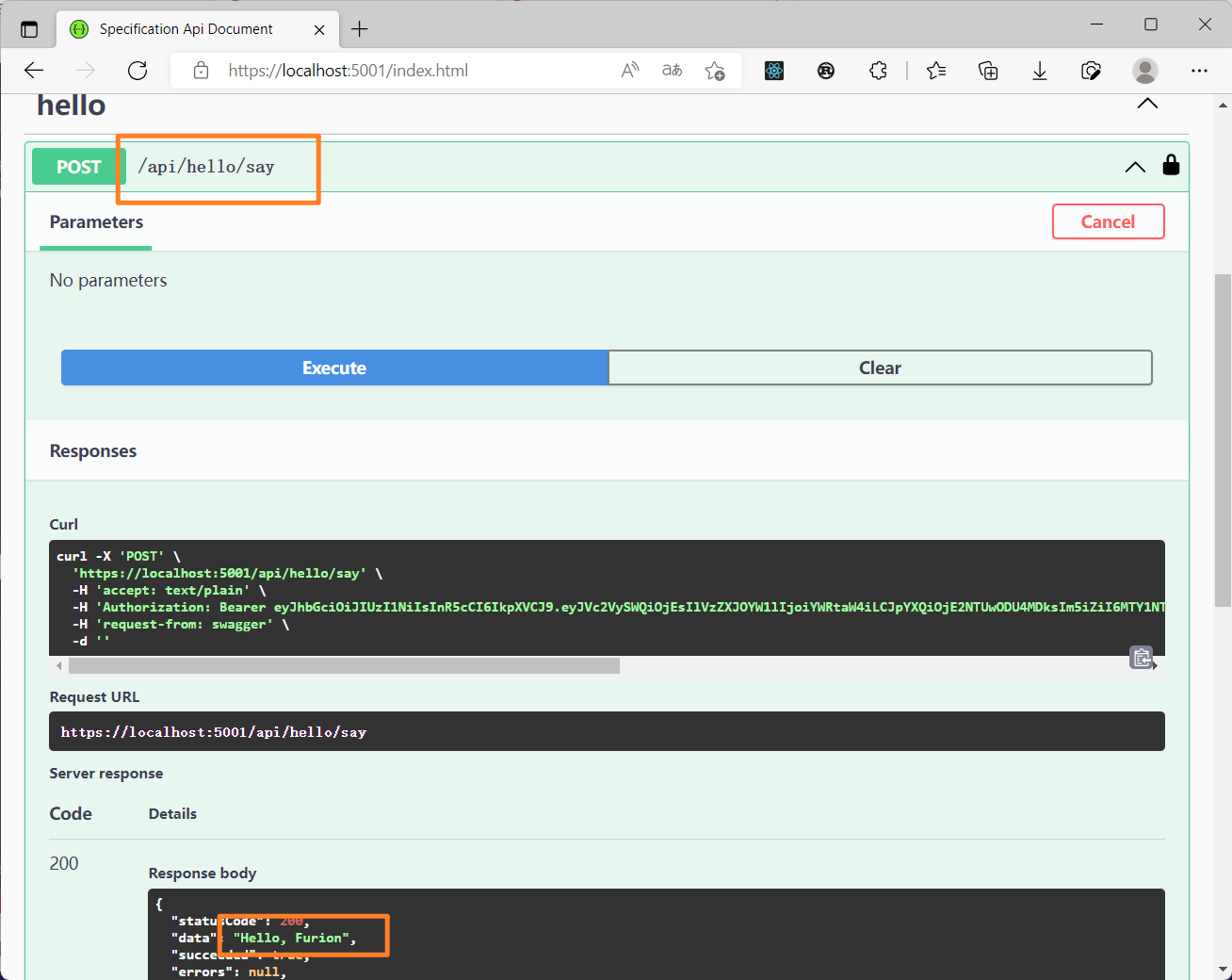
2.1.7 Serve.Run()
更多配置
2.1.7.1 配置默认启动地址/端口
默认情况下,创建的 Web
主机端口为 5000/5001
端口,如需自定义配置,可通过第一个参数配置:
Serve.Run("https://localhost:8080");
// 或
Serve.Run(RunOptions.Default, "https://localhost:8080");
同时也支持 dotnet run
和 dotnet watch run
指定:
dotnet run --urls https://localhost:8080
# watch 方式
dotnet watch run --urls https://localhost:8080
也可以通过 ConfigureBuilder
方式配置:
// .NET6+
Serve.Run(RunOptions.Default.ConfigureBuilder(builder =>
{
builder.WebHost.UseUrls("https://localhost:8080"); // 也可以通过 builder.Configuration 读取 urls 配置
}));
// .NET5
Serve.Run(RunOptions.ConfigureWebDefaults(webHostBuilder =>
{
return webHostBuilder.UseUrls("https://localhost:8080");
}));
localhost
和多端口建议使用 *
代替 localhost
,这样可以自适应主机地址,多个端口使用 ;
分割,结尾无需 ;
json
方式配置如需通过配置文件配置端口,需两个该步骤:
- 编辑控制台启动项目
.csproj
文件,修改Project
节点为:
<Project Sdk="Microsoft.NET.Sdk.Web">
也就是在原来的 Sdk
中添加 .Web
即可。
- 在控制台启动项目中添加
Properties
文件夹并在此文件夹中创建launchSettings.json
文件,同时写入以下内容:
{
"$schema": "http://json.schemastore.org/launchsettings.json",
"profiles": {
"启动项目名称": {
"commandName": "Project",
"launchBrowser": true,
"launchUrl": "",
"applicationUrl": "https://localhost:8080;http://localhost:8081",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
}
}
}
除了 launchsettings.json
的方式,还可以在 appsettings.json
简单配置
{
"Urls": "http://localhost:8081"
}
- 还可以获取随机空闲端口启动
以下内容仅限 Furion 4.8.7.29 +
版本使用。
通过 Serve.IdleHost
静态属性可以获取随机的 Web
主机地址(端口),如:http://localhost:随机端口
。
Serve.Run(urls: Serve.IdleHost.Urls);
// 完整返回值
var idleHost = Serve.IdleHost;
var port = idleHost.Port; // => 44322
var urls = idleHost.Urls; // => http://localhost:44322
var ssl_urls = idleHost.SSL_Urls; // => https://localhost:44322,Furion 4.8.7.43+ 支持
// 指定主机名,Furion 4.8.7.43+ 支持
var idleHost = Serve.GetIdleHost("furion.baiqian.ltd"); // 不传参数默认 localhost
var port = idleHost.Port; // => 44322
var urls = idleHost.Urls; // => http://furion.baiqian.ltd:44322
var ssl_urls = idleHost.SSL_Urls; // => http://furion.baiqian.ltd:44322
2.1.7.2 便捷服务注册
以下内容仅限 Furion 4.8.0 +
版本使用。
Serve.Run(additional: services =>
{
services.AddRemoteRequest();
});
// 通用泛型主机方式
Serve.RunGeneric(additional: services =>
{
services.AddRemoteRequest();
});
// 还可以省去 additional
Serve.Run(services =>
{
services.AddRemoteRequest();
});
// 通用泛型主机方式
Serve.RunGeneric(services =>
{
services.AddRemoteRequest();
});
2.1.7.3 自定义配置
传入 RunOptions
对象相当于自由定义和控制,也就是除了默认集成了 Furion
以外,没有注册任何功能。
- 仅集成
Furion
的默认配置
Serve.Run(RunOptions.Default);
- 配置更多服务/中间件
Serve.Run(RunOptions.Default
.ConfigureBuilder(builder =>
{
builder.Services.AddControllers()
.AddInject();
})
.Configure(app =>
{
app.UseRouting();
app.UseInject(string.Empty);
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}));
WebComponent
方式
以下内容仅限 Furion 4.3.5 +
版本使用。
Serve.Run(RunOptions.Default
.AddWebComponent<XXXWebComponent>());
public class XXXWebComponent : IWebComponent
{
public void Load(WebApplicationBuilder builder, ComponentContext componentContext)
{
// ....
}
}
2.1.7.4 Serve.Run
和 Startup
最佳组合
默认情况下 Serve.Run()
内置了 跨域
,控制器
,路由
,规范化结果
、静态文件
服务/中间件。适合快速开始项目和编写测试代码。
但不能对这些已注册服务/中间件进行自定义配置,这时只需要配置 RunOptions
属性/方法即可,如:
Serve.Run(RunOptions.Default
.ConfigureBuilder(...)
.Configure(..));
**但把所有服务/中间件都放在 Program.cs
中好吗?**答案是不好的,因为会导致后续迁移代码维护代码造成了一些困扰。
所以 Furion
推荐下面更加灵活且易维护的方式,Program.cs
只需一句话即可:
Furion 3.7.3+
官方提供了非常灵活方便的组件化启动配置服务。
推荐使用 《3.1 组件化启动》代替 AppStartup
方式功能。
Serve.Run(RunOptions.Default);
然后添加自定义 Startup.cs
文件,代码如下:
using Furion;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.DependencyInjection;
namespace HelloFurion;
public class Startup : AppStartup
{
public void ConfigureServices(IServiceCollection services)
{
// ....
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// ....
}
}
正常情况下,自定义 Startup.cs
文件应该放在独立的 YourProject.Web.Core
层或其他层。
2.1.7.5 更多配置
如配置 WebHost
...
Serve.Run(RunOptions.Default
.ConfigureBuilder(builder => {
builder.WebHost.....
}));
2.1.8 支持 Furion
所有功能
Serve.Run()
看似非常简单,实则非常灵活,而且支持 Furion
和 .NET
所有功能。
2.1.8.1 添加 appsettings.json
创建 appsettings.json
文件,并设置 属性
为 如果较新则复制
和 内容
(生成操作)
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information",
"Microsoft.EntityFrameworkCore": "Information"
}
},
"AllowedHosts": "*"
}
在代码中读取配置:
using Furion;
Serve.Run();
[DynamicApiController]
public class HelloService
{
public string Say()
{
return "Hello, Furion " + App.Configuration["Logging:LogLevel:Default"];
}
}
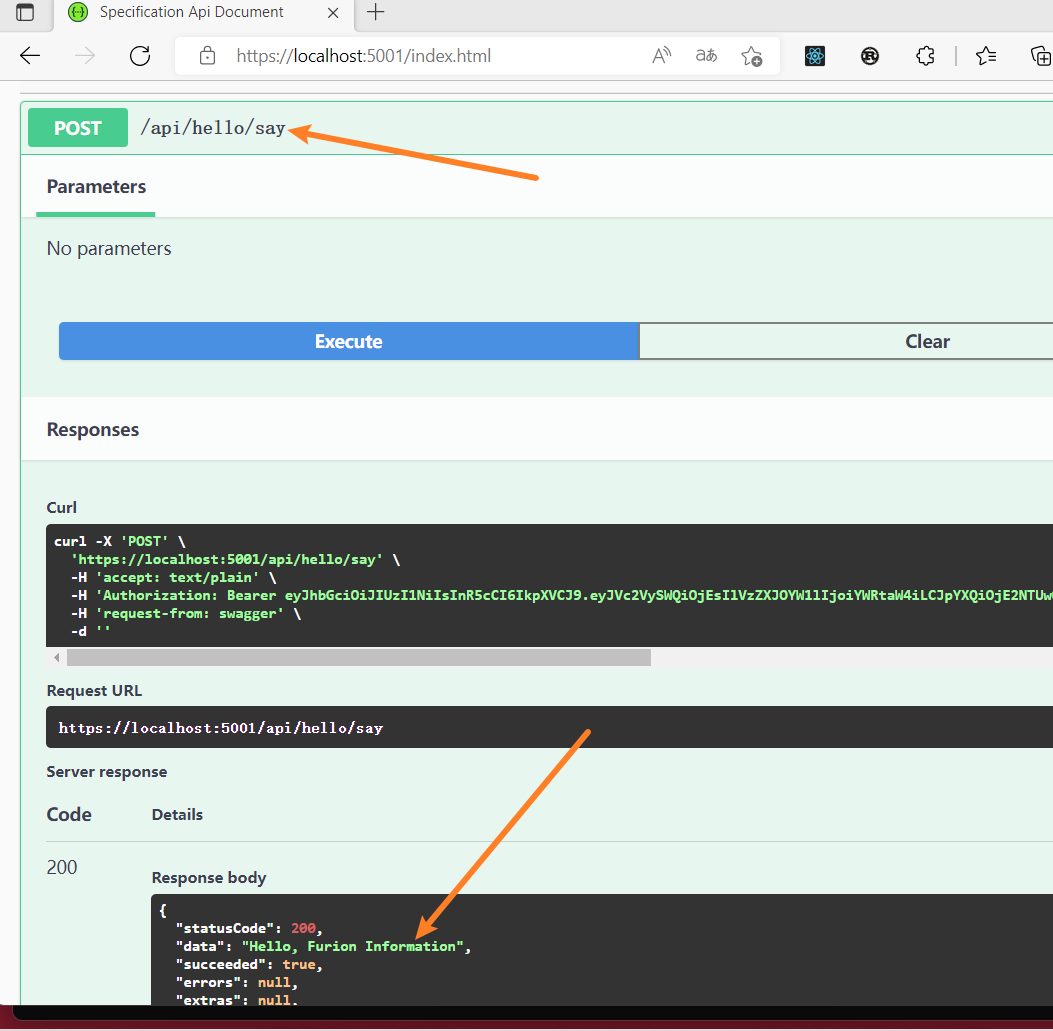
2.1.8.2 添加自定义 Startup
在 Furion
中可以派生自 AppStartup
可以实现更多配置,如:
Serve.Run();
如果您想自己配置 Web
项目服务,可通过 Serve.Run(RunOptions.Default);
方式,因为 Serve.Run()
已经包含了常用的 Web
可能会提示重复注册错误。
using Furion;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.DependencyInjection;
namespace HelloFurion;
public class MyStartup : AppStartup
{
public void ConfigureServices(IServiceCollection services)
{
Console.WriteLine("调用服务注册啦~~");
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
Console.WriteLine("调用中间件注册啦");
}
}
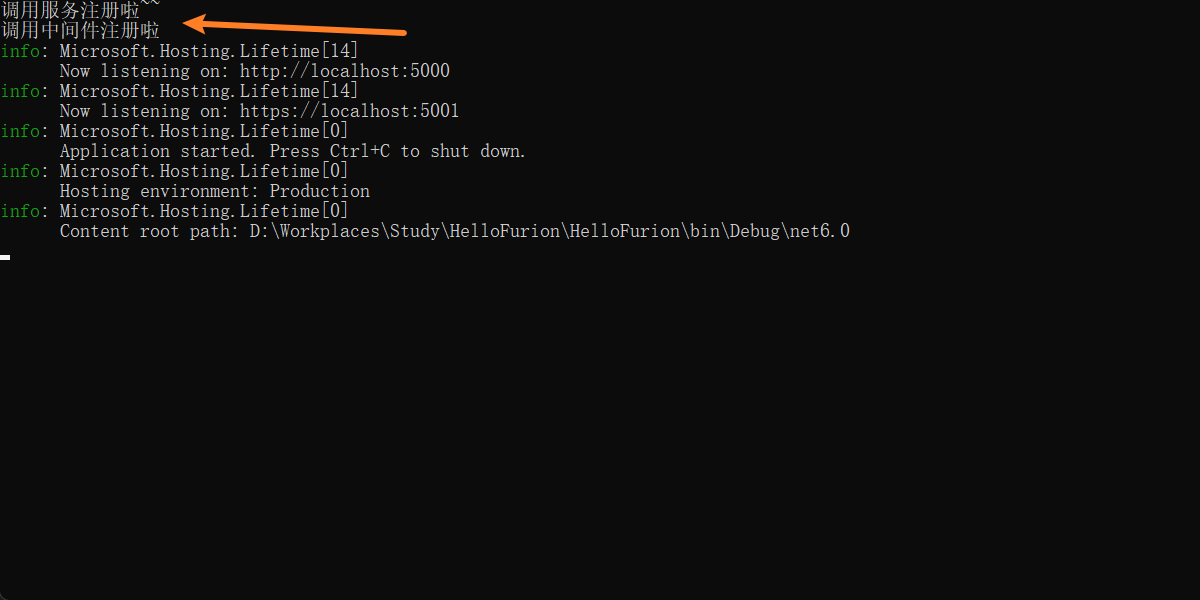
2.1.8.3 将控制台项目变成 Web
项目
只需要编辑 .csproj
文件,将第一行 Project
节点的 Sdk
<Project Sdk="Microsoft.NET.Sdk">
修改为:
<Project Sdk="Microsoft.NET.Sdk.Web">
即可完成转 换,实际上只是追加了 .Web
。
2.1.8.4 添加 args
启动参数
以下内容仅限 Furion 4.2.4 +
版本使用。
Serve.Run(args: args);
Serve.Run(RunOptions.Default.WithArgs(args));
Serve.Run(RunOptions.Main(args));
2.1.8.5 还没看够?
是不是非常强大啊,Serve.Run()
虽然简单,但是 100% 支持 Furion
和 .NET
所有功能。尽情去体验吧!
2.1.9 RunOptions
,LegacyRunOptions
和 GenericRunOptions
Serve.Run
提供 了 RunOptions
,LegacyRunOptions
和 GenericRunOptions
重载参数类型,他们的主要区别:
RunOptions
:使用的是WebApplication
方式,创建Web
主机优先推荐方式LegacyRunOptions
:使用的是Host
方式,但默认配置了Web
主机GenericRunOptions
:使用的是Host
方式,通用类型主机,可用于WorkerService
LegacyRunOptions
配置例子:
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.DependencyInjection;
Serve.Run(LegacyRunOptions.Default
// 配置 Web 主机
.ConfigureWebDefaults(builder => builder.ConfigureServices(services =>
{
// ...
})
.Configure(app =>
{
// ...
});
})
// 配置 Host 主机
.ConfigureBuilder(builder => builder....));
GenericRunOptions
配置例子:
Serve.Run(GenericRunOptions.Default
// 配置 Host 主机
.ConfigureBuilder(hostBuilder => hostBuilder....);
更多发布命令说明可查阅微软官方文档 https://docs.microsoft.com/en-us/dotnet/core/tools/dotnet-publish
2.1.10 在 WinForm/WPF/Console
中使用
在 WinForm
或 WPF
或 Console
中使用,请确保 Serve.RunNative()
在应用程序之前初始化:
2.1.10.1 WinForm
初始化
namespace WinFormsApp2;
internal static class Program
{
[STAThread]
static void Main()
{
Serve.RunNative(); // Furion 4.8.7.26+ 支持
ApplicationConfiguration.Initialize();
Application.Run(new Form1());
}
}
Serve.RunNative
端口占用问题默认情况下,Serve.RunNative()
会启动一个迷你小型 Web
主机,默认端口为 5000
,如无需 Web
功能可配置 includeWeb
关闭,如:
// 关闭迷你 Web 主机功能
Serve.RunNative(includeWeb: false);
// 或更换迷你 Web 主机端口
Serve.RunNative(urls: "http://localhost:8080");
// 还可以完全自定义配置 Web 主机
Serve.RunNative(RunOptions.Default);
// 还可以随机获取一个空闲主机端口(推荐)
Serve.RunNative(urls: Serve.IdleHost.Urls);
2.1.10.2 WPF
初始化
namespace WpfApp1;
public partial class App : Application
{
public App()
{
Serve.RunNative(); // Furion 4.8.7.23+
}
}
Serve.RunNative
端口占用问题默认情况下,Serve.RunNative()
会启动一个迷你小型 Web
主机,默认端口为 5000
,如无需 Web
功能可配置 includeWeb
关闭,如:
// 关闭迷你 Web 主机功能
Serve.RunNative(includeWeb: false);
// 或更换迷你 Web 主机端口
Serve.RunNative(urls: "http://localhost:8080");
// 还可以完全自定义配置 Web 主机
Serve.RunNative(RunOptions.Default);
// 还可以随机获取一个空闲主机端口(推荐)
Serve.RunNative(urls: Serve.IdleHost.Urls);
2.1.10.3 Console
初始化
Console.WriteLine("Hello World");
Serve.RunNative(); // Furion 4.8.7.23+
Console.ReadKey();
2.1.10.4 注册服务
如果想注册服务,支持以下两种方式:
Serve.RunNative
方式
Serve.RunNative(services =>
{
services.AddRemoteRequest();
});
Startup.cs
方式
// 在迷你 Web 主机基础上自定义
Serve.RunNative();
// 或完全自定义
Serve.RunNative(RunOptions.Default);
using Furion;
using Microsoft.Extensions.DependencyInjection;
namespace YourProject;
public class YourStartup : AppStartup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddRemoteRequest();
}
}
2.1.11 静默启动
默认情况下,Serve.Run()
使用阻塞线程方式启动,但有些时候我们不希望阻塞现有的代码,可使用静默启动的方式:
Serve.Run(silence: true);
Console.WriteLine("Hello, World!");
Console.ReadKey();
也可以通过 RunOptions
,LegacyRunOptions
或 GenericRunOptions
方式,如:
// RunOptions 方式
Serve.Run(RunOptions.DefaultSilence);
// LegacyRunOptions 方式
Serve.Run(LegacyRunOptions.DefaultSilence);
// GenericRunOptions 方式
Serve.Run(GenericRunOptions.DefaultSilence);
2.1.12 .NET5
模式找不到 Views
视图路径
由于 .NET5
必须在使用 .UseStartup<>
配置启动项,所以 Serve.Run()
模式会提示找不到 Views
视图路径,这时候只需要在启动目录创建 Startup.cs
文件并通过泛型方式指定即可,如:
using Microsoft.AspNetCore.Builder;
using Microsoft.Extensions.DependencyInjection;
namespace YourProject.Web.Entity
{
public class Startup
{
public void ConfigureServices(IServiceCollection _)
{
}
public void Configure(IApplicationBuilder _)
{
}
}
}
将 Startup
类通过 Serve.Run
泛型指定:
Serve.Run<Startup>(LegacyRunOptions.Default);
2.1.13 反馈与建议
给 Furion 提 Issue。